About
Description
smFilteredPagination is a jQuery pagination plugin that has been designed to be more flexible than existing plugins by using counts instead of wrappers to define pages. This allows for items to be filtered dynamically without changing the number of results per page.
Explanation
Consider the following design patterns. One that uses wrappers around items to define pages, where results are then filtered. It can get quite hairy very quickly as the counts per page will be incosistent and confusing to the end-user. What makes smFilteredPagination a better alternative is in the way it handles pages, using calculations based on classes. The same example without pages is shown on the right. This is how smFilteredPagination works.
<!-- This example would be showing page 2, displaying 1 item. -->
<div class="page hide">
<div class="item"></div>
<div class="item"></div>
<div class="item"></div>
</div>
<div class="page">
<div class="item filtered"></div>
<div class="item"></div>
<div class="item filtered"></div>
</div>
<div class="page hide">
<div class="item filtered"></div>
<div class="item"></div>
<div class="item"></div>
</div>
<!-- not very flexible -->
<!-- Showing page 2, displaying 3 items, 2 came from "page 3". -->
<div class="results">
<div class="item hide"></div>
<div class="item hide"></div>
<div class="item hide"></div>
<div class="item filtered hide"></div>
<div class="item"></div>
<div class="item filtered hide"></div>
<div class="item filtered hide"></div>
<div class="item"></div>
<div class="item"></div>
</div>
<!-- much more flexible (how smFilteredPagination works) -->
The hidden class is used to hide any items outside the currently viewed "slice". In addition to the hidden class, you may add a list of classes that can be used as filters to dynamically filter items from the pagination.
smFilteredPagination aims to be a simple, highly configurable and flexible javascription pagination solution.
Please read the source code and check out the demos for more information.
Required Files
- jQuery 1.4+
- jquery.smFilteredPagination.js
- jquery.smFilteredPagination.css
Add them to your document like such:
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.6.4/jquery.min.js"></script>
<script src="js/jquery.smFilteredPagination.js"></script>
<link rel="stylesheet" href="css/jquery.smFilteredPagination.css" type="text/css" media="all" />
Download
All source is available for download at github.com/saltermitchell.
This documentation is a work in progress, please read the page source if you're having trouble.
Usage Examples
Example 1: Default
$(function(){ // document ready
pagination1 = new $.smFilteredPagination($("#pagination-1"));
});
<div id="pagination-1" class="example-pagination">
<div class="results">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
...
<div class="item">Item 30</div>
<div class="item">Item 31</div>
<div class="item">Item 32</div>
<div>
<div>
This is the minimal example of pagination which uses the default options specified at the bottom of this document.
Example 2: # Per Page and Live Modification to Items
$(function(){ // document ready
pagination2 = new $.smFilteredPagination($("#pagination-2"), {
pagerHeader: "pagerHeader2",
pagerFooter: "pagerFooter2",
itemsPerPage: 20
});
// using public methods
$("#p2-per-page").change(function() {
pagination2.setItemsPerPage($(this).val());
});
// toggle filtering of even rows
$("#p2-filter-even").click(function() {
if ($(this).hasClass("off")) {
$(this).removeClass("off").addClass("on");
$("#pagination-2 div.item:odd").addClass("filtered");
$("span",this).text("on");
} else {
$(this).removeClass("on").addClass("off");
$("#pagination-2 div.item.filtered").removeClass("filtered");
$("span",this).text("off");
}
pagination2.rebuild();
return false; // prevent default
});
$("#p2-add").click(function() {
// this can be dynamic, consider "items.php?start="+$("last-id").val()+"&count=10"
pagination2.addItems("examples/ajax-items.html");
return false; // prevent default
});
});
<div id="pagination-2" class="example-pagination">
<div class="results">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
...
<div class="item">Item 30</div>
<div class="item">Item 31</div>
<div class="item">Item 32</div>
<div>
<div>
This example shows how to use public methods with useful scenarios, such as updating the page count and adding/removing filters. Try increasing the count to 6 or 10 and them "filter even rows". Remove the filter and change to 40 to see pagination hide itself if the pageCount = 1.
Example 3: New Pager Names and scrollToTopOnChange
$(function(){ // document ready
pagination3 = new $.smFilteredPagination($("#pagination-3"), {
pagerItems: ".pic",
showPagerHeader: false,
showPagerFooter: true,
pagerFooter: "pagerFooter3",
textFirst: "<<",
textPrev: "<",
textNext: ">",
textLast: ">>",
itemsPerPage: 5,
scrollToTopOnChange: true
});
});
<div id="pagination-3" class="example-pagination">
<div class="results">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
...
<div class="item">Item 30</div>
<div class="item">Item 31</div>
<div class="item">Item 32</div>
<div>
<div>
This example utilizes some of the options to change how pagination appears and behaves.
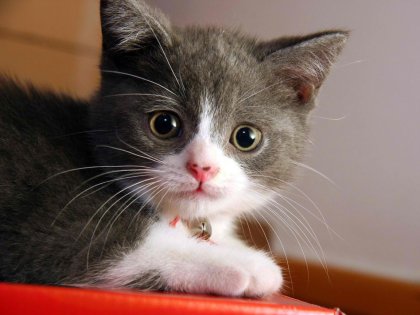
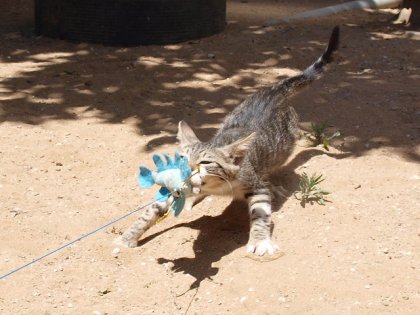
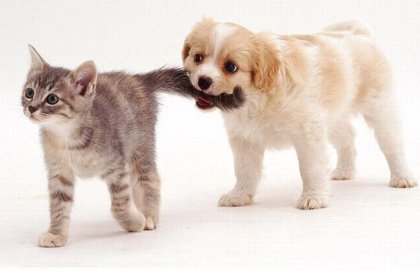
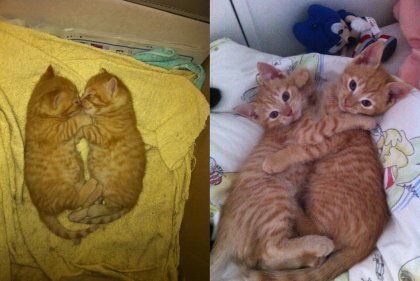
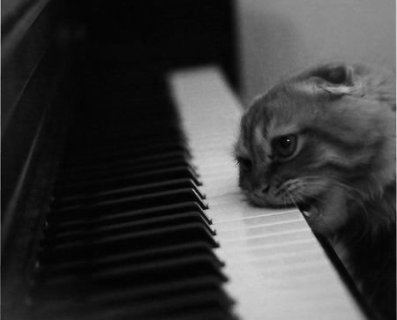
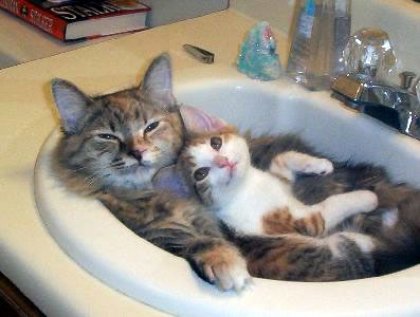
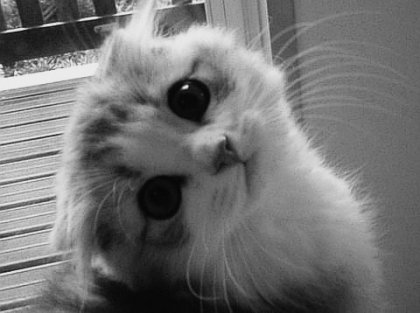
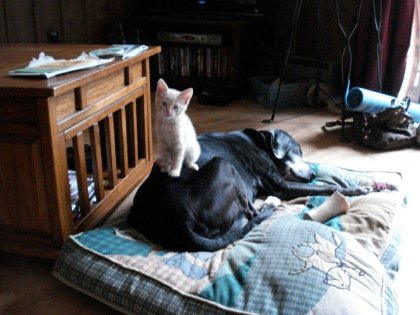
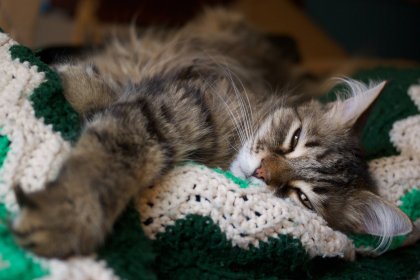
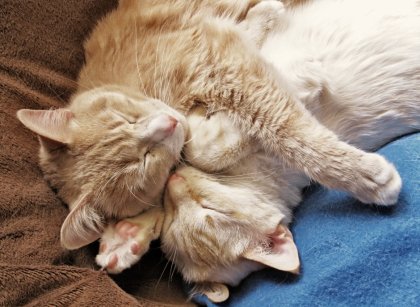
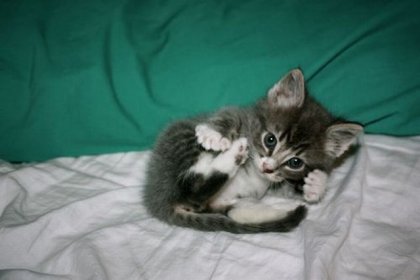
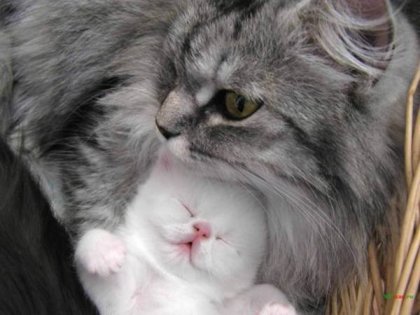
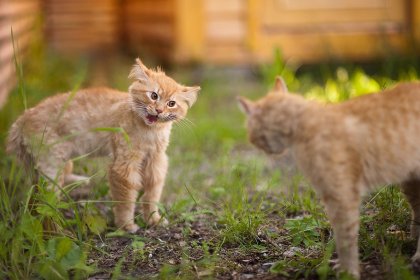
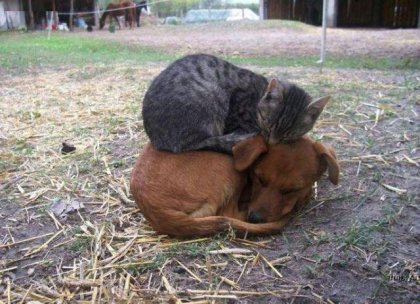
Options
The following plugin options are configurable, they are shown below with their defaults.
options = {
pagerItems: "div.item", // item class
pagerItemsWrapper: "div.results", // item wrapping class or id
pagerClass: "pagination", // pager class
pagerHeader: "pagerHeader", // header pager id
pagerFooter: "pagerFooter", // footer pager id
itemsPerPage: 6, // items per page
itemsInPager: 6, // max items displayed in pager
itemsInPagerEdge: 2, // max items displayed in pager edges
itemsInPagerContinued: "...", // text to display when page numbers are hidden
showPagerHeader: true, // show header pager?
showPagerFooter: true, // show footer pager?
showFirst: true, // show "first"
showPrev: true, // show "prev"
showNext: true, // show "next"
showLast: true, // show "last"
showOnZero: false, // show pagination on zero results
showOnOne: false, // show pagination on one page of results
textFirst: "First", // "first" text
textPrev: "Previous", // "prev" text
textNext: "Next", // "next" text
textLast: "Last", // "last" text
hiddenClass: "hide", // name of hidden class
filteredClassList: ".filtered", // comma separated list of filtered classes
scrollToTopOnChange: false, // Scroll to the top of the page on change.
handleLocationHash: true, // handle page numbers with the location hash, page.php#5
locationHashHandler: function(pl) { // function to handle the page hash on page load
var page = location.hash.split("#")[1];
if (isNumber.test(page)) { pl.setCurrentPage(page); }
},
locationHashUpdate: function(page) {// function to handle the page hash on update
location.hash = page;
},
insertPagerHeader: function(el) { // insertPagerHeader function, automatically inserts pagerHeader if not found and show is true
var pagerContents = '<div id="' + this.pagerHeader + '" class="' + this.pagerClass + '"></div>';
if ($("#"+this.pagerHeader).length) { $("#"+this.pagerHeader).html(pagerContents); }
else { $(el).prepend(pagerContents); }
},
insertPagerFooter: function(el) { // insertPagerFooter function, automatically inserts pagerFooter if not found and show is true
var pagerContents = '<div id="' + this.pagerFooter + '" class="' + this.pagerClass + '"></div>';
if ($("#"+this.pagerFooter).length) { $("#"+this.pagerFooter).html(pagerContents); }
else { $(el).append(pagerContents); }
}
}
Public Methods
The following public methods are available through the initialized object.
- setItemsPerPage(n) - sets the # of items per page
- setCurrentPage(n) - sets the current page
- getItemsPerPage() - returns the # of items per page
- getCurrentPage() - returns the current page
- getPageCount() - returns the page count
- addItems(file) - adds more items with ajax
- rebuild() - rebuilds the pagination
Contact
If you have a question, concern, request or otherwise need to contact me, you may email tyler.mulligan@saltermitchell.com. Thats: Tyler [dot] Mulligan [at] Salter Mitchell (dot) com.
Last Updated: 11/03/2011